Android Chat Bubble 9 Patch Download
Posted : admin On 18.12.2020- Android Chat Bubble 9 Patch Download Torrent
- Android Chat Bubble 9 Patch Download Pc
- Android Chat Bubble 9 Patch Download Free
- Android Chat Bubble 9 Patch Download Windows 7
Image: Working Friendly Chat app.
- This Speech Bubble Thin - Android Chat Bubble Png is high quality PNG picture material, which can be used for your creative projects or simply as a decoration for your design & website content. Speech Bubble Thin - Android Chat Bubble Png is a totally free PNG image with transparent background and its resolution is 945x744.
- $ ninepatch wrap ninepatchbubble.9.png imagetowrap.png wrapped.png Slice the 9patch into tiles: $ ninepatch slice ninepatchbubble.9.png./outputdir Interactive viewer. Interactively resize and preview an image in a Tkinter viewer: $ ninepatchviewer ninepatchbubble.9.png or just: $ ninepatchviewer without arguments to see the demo image.
Test your apps for compatibility with Android 9. Just download a device system image, install your current app, and test in areas where behavior changes may affect the app. Update your code and publish, using the app's current platform targeting. Bubbles for Android. Bubbles for Android is an Android library to provide chat heads capabilities on your apps. With a fast way to integrate with your development. How to use Configuring your project dependencies. Add the library dependency in your build.gradle file. 9 Patch images contain an index of which piece is what by adding a 1px border to the image. The colors in the border determine if a piece is static (doesn't scale), it stretches, or it repeats. For more details see the wiki page: What Are 9 Patch Images. How to Create 9 Patch Images. To create a 9 patch image you need to start with a.png or a. Don't be jealous of iMessage. Here's how to use Google's fancy Chat feature on Android phones. If you're not using Google Chat's enhanced messaging features, now's the time to learn them all.
Welcome to the Friendly Chat codelab. In this codelab, you'll learn how to use the Firebase platform to create a chat app on Android.
What you learn to do
- Allow users to sign in.
- Sync data using the Firebase Realtime Database.
- Store binary files in Firebase
What you need
- Android Studio version 3.4+.
- Sample code.
- A test device with Android 2.3+ and Google Play services 9.8 or later, or an Emulator with Google Play services 9.8 or later
- If using a device, a connection cable.
Clone the GitHub repository from the command line: Download instagram for android 5.1.1.
The 'friendlychat-android' repository contains two directories:
- build-android-start—Starting code that you build upon in this codelab.
- build-android—Completed code for the finished sample app.
Note: If you want to run the finished app, you have to create a project in the Firebase console corresponding to the package name and SHA1. See Create Firebase Console Project for the command. Also you will have to enable Google as an Auth Provider; do this in the Authentication section of the Firebase console.
From Android Studio, select the build-android-start
directory ( ) from the sample code download (File > Open > ../codelab-friendlychat-android/build-android-start).
You should now have the android-start project open in Android Studio. If you see a warning about a google-services.json file missing, don't worry. It will be added in the next step.
Add Firebase to the project
- Go to the Firebase console.
- Select Add project.
- Select or enter a Project name.
- Follow the remaining setup steps in the Firebase console, then click Create project (or Add Firebase, if you're using an existing Google project).
- From the overview screen of your new project, click the Android icon to launch the setup workflow.
- Enter the codelab's package name:
com.google.firebase.codelab.friendlychat
- Enter the SHA1 of your signing keystore. If you are using the standard debug keystore, use the command below to find the SHA1 hash:
Note: Your debug keystore, usually called 'debug.keystore', is typically located at
$HOME/.android/debug.keystore. If this file does not exist, Android Studio will create it for you the first time you run any app.
See here for more detail on finding SHA1.
Add google-services.json file to your app
After adding the package name and SHA1 and selecting Register**, Click Download google-services.json** to obtain your Firebase Android config file then copy the google-services.json file into the *app
* directory in your project. After the file is downloaded you can **Skip** the next steps shown in the console (they've already been done for you in the build-android-start project).
Add google-services plugin to your app
The google-services plugin uses the google-services.json file to configure your application to use Firebase. The following line should already be added to the end of the build.gradle file in the app
directory of your project (check to confirm):
Sync your project with gradle files
To be sure that all dependencies are available to your app, you should sync your project with gradle files at this point. Select File > Sync Project with Gradle Files from the Android Studio toolbar.
Now that you have imported the project into Android Studio and configured the google-services
plugin with your JSON file, you are ready to run the app for the first time. Connect your Android device, and click Run ( )in the Android Studio toolbar.
The app should launch on your device. At this point, you should see an empty message list, and sending and receiving messages will not work. In the next section, you authenticate users so they can use Friendly Chat.
Let's require a user to sign in before reading or posting any Friendly Chat messages.
Firebase Realtime Database Rules
Access to your Firebase Database is configured by a set of rules written in a JSON configuration language.
Go to your project in the Firebase console and select Database. Select the Realtime Database option (not Cloud Firestore). If prompted for security rules, with choices to start in either test mode or locked mode, choose locked mode. Once the default rules are established, select the Rules tab and update the rules configuration with the following:
Click 'Publish' to publish the new rules.
For more information on how this works (including documentation on the 'auth' variable) see the Firebase security documentation.
Configure Authentication APIs
Before your application can access the Firebase Authentication APIs on behalf of your users, you will have to enable it
- Navigate to the Firebase console and select your project
- Select Authentication
- Select the Sign In Method tab
- Toggle the Google switch to enabled (blue)
- Set a support email.
- Press Save on the resulting dialog
If you get errors later in this codelab with the message 'CONFIGURATION_NOT_FOUND', come back to this step and double check your work.
Add Firebase Auth dependency
The firebase-auth SDK allows easy management of authenticated users of your application. Confirm the existence of this dependency in your app/build.gradle
file.
app/build.gradle
Add the Auth instance variables in the MainActivity
class under the // Firebase instance variables
comment:
MainActivity.java (instance variable)
Check for current user
Now let's modify MainActivity.java
to send the user to the sign-in screen whenever they open the app and are unauthenticated.
Add the following to the onCreate
method after mUsername
has been initialized:
Android Chat Bubble 9 Patch Download Torrent
MainActivity.java
Then add a new case to onOptionsItemSelected()
to handle the sign out button:
MainActivity.java
Now we have all of the logic in place to send the user to the sign-in screen when necessary. Next we need to implement the sign-in screen to properly authenticate users.
Implement the Sign-In screen
Open the file SignInActivity.java
. Here a simple Sign-In button is used to initiate authentication. In this step you will implement the logic to Sign-In with Google, and then use that Google account to authenticate with Firebase.
Add an Auth instance variable in the SignInActivity
class under the // Firebase instance variables
comment:
SignInActivity.java
Then, edit the onCreate()
method to initialize Firebase in the same way you did in MainActivity
:
SignInActivity.java
Next, initiate signing in with Google. Update SignInActivity
's onClick
method to look like this:
SignInActivity.java
Add the required signIn method that actually presents the user with the Google Sign-In UI.
SignInActivity.java
Next, add the onActivityResult
method to SignInActivity
to handle the sign in result. If the result of the Google Sign-In was successful, use the account to authenticate with Firebase.
SignInActivity.java
Add the required firebaseAuthWithGoogle
method to authenticate with the signed in Google account:
SignInActivity.java
That's it! You've implemented authentication using Google as an Identity Provider in just a few method calls and without needing to manage any server-side configuration.
Test your work
Run the app on your device. You should be immediately sent to the sign-in screen. Tap the Google Sign-In button. You should then be sent to the messaging screen if everything worked well.
Import Messages
- In your project in Firebase console, select Database on the left navigation menu.
- Select Realtime Database option.
Note: If you are presented with database creation option, please select Realtime database create option and apply the rules as done in previous step.
- In the overflow menu of the Data tab, select Import JSON.
- Browse to the initial_messages.json file in the root of the cloned repository, and select it.
- Click Import.
Note: This replaces any data currently in your database.
After importing the JSON file, your database should look like this:
Add Firebase Realtime Database and Firebase Storage dependencies
In the dependencies block of the app/build.gradle file, the following dependencies should be included. For this codelab, they are already added for convenience; confirm this by looking in the app/build.gradle file:
Dependency in app/build.gradle
Synchronize messages
In this section we add code that synchronizes newly added messages to the app UI by:
- Initializing the Firebase Realtime Database and adding a listener to handle changes made to the data.
- Updating the
RecyclerView
adapter so new messages will be shown. - Adding the Database instance variables with your other Firebase instance variables in the
MainActivity
class:
MainActivity.java
Modify your MainActivity's onCreate
method by replacing mProgressBar.setVisibility(ProgressBar.INVISIBLE);
with the code defined below. This code initially adds all existing messages and then listens for new child entries under the messages path in your Firebase Realtime Database. It adds a new element to the UI for each message:
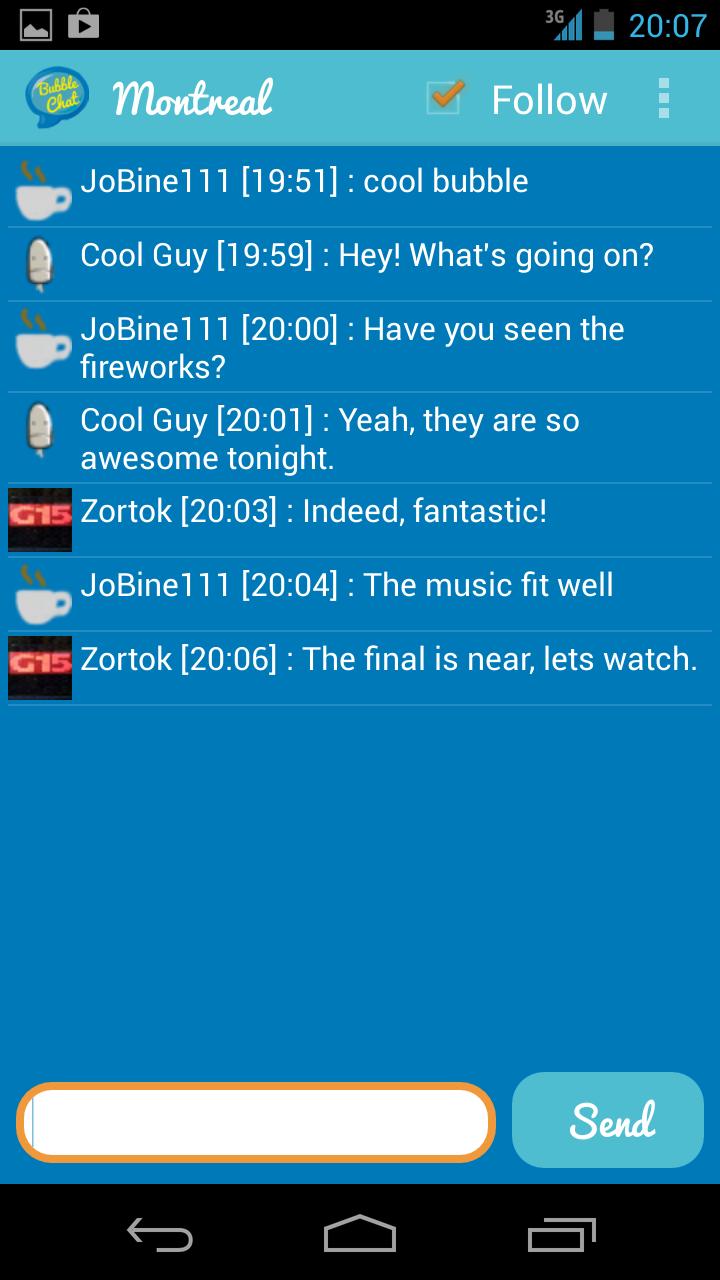
MainActivity.java
Appropriately start and stop listening for updates from Firebase Realtime Database. Update the onPause
and onResume
methods in MainActivity
as shown below.
MainActivity.java
Test message sync
- Click Run ( ).
- Add new messages directly in the Database section of the Firebase console. Confirm that they show up in the Friendly-Chat UI.
- Navigate to the Database section of the Firebase console. From the Data tab, select the ‘+' sign on the messages element.
- Give the new element a name of -ABCD (note the ‘-' sign) and leave the value empty for now.
- Select the ‘+' sign on the -ABCD element
- Give the new element a name of 'name' and value of 'Mary'
- Select the ‘+' sign on the -ABCD element again
- Give the new element a name of 'text' and value of 'hello'
- Select Add
Congratulations, you just added a realtime database to your app!
Implement text message sending
In this section, you will add the ability for app users to send text messages. The code snippet below listens for click events on the send button, creates a new FriendlyMessage
object with the contents of the message field, and pushes the message to the database. The push()
method adds an automatically generated ID to the pushed object's path. These IDs are sequential which ensures that the new messages will be added to the end of the list.
Update the onClick
method of mSendButton
in the onCreate
method in the MainActivity
class. This code is at the bottom of the onCreate
method already. Update the onClick
body to match the code below:
MainActivity.java
Implement image message sending
In this section, you will add the ability for app users to send image messages. Creating an image message is done with these steps:
- Select image
- Handle image selection
- Write temporary image message to the RTDB (Realtime Database)
- Begin to upload selected image
- Update image message URL to that of the uploaded image, once upload is complete
Select Image
To add images this codelab uses Cloud Storage for Firebase. Cloud Storage is a good place to store the binary data of your app.
In the Firebase console select Storage in the left navigation panel. Then click Get Started to enable Cloud Storage for your project. Continue following the steps in the prompt, using the suggested defaults.
Android Chat Bubble 9 Patch Download Pc
With the following code snippet you will allow the user to select an image from the device's local storage. Update the onClick
method of mAddMessageImageView
in the onCreate
method in the MainActivity
class. This code is at the bottom of the onCreate
method already. Update the onClick
body to match the code below:
MainActivity.java
Android Chat Bubble 9 Patch Download Free
Handle image selection and write temp message
Once the user has selected an image, a call to the MainActivity
's onActivityResult
will be fired. This is where you handle the user's image selection. Using the code snippet below, add the onActivityResult
method to MainActivity
. In this function you will write a message with a temporary image url to the database indicating the image is being uploaded.
MainActivity.java
Upload image and update message
Add the method putImageInStorage
to MainActivity
. It is called in onActivityResult
to initiate the upload of the selected image. Once the upload is complete you will update the message to use the appropriate image.
MainActivity.java
Test Sending Messages
- Click the Run button.
- Enter a message and hit the send button, the new message should be visible in the app UI and in the Firebase console.
- Tap the '+' image to select an image from your device. The new message should be visible first with a placeholder image, and then with the selected image once the image upload is complete. The new message should also be visible in the Firebase console, as an object in the Database and as a blob in Storage.
You have used Firebase to easily build a real-time chat application.
What we've covered
- Firebase Authentication
- Firebase Realtime Database
- Cloud Storage for Firebase
Next Steps
- Try the Grow Friendly Chat codelab, to learn how to grow the app you just built.
- Use Firebase in your own Android app.
Learn More
The Draw 9-patch tool is a WYSIWYG editor included in Android Studio that allows you to create bitmap images that automatically resize to accommodate the contents of the view and the size of the screen. Selected parts of the image are scaled horizontally or vertically based on indicators drawn within the image.
For an introduction to NinePatch graphics and how they work, please readthe section about NinePatch Drawables in theCanvas and Drawablesdocument.
Figure 1. A NinePatch image in Android Studio's Draw 9-patch tool.
Here's a quick guide to creating a NinePatch graphic using the Draw 9-patch tool in Android Studio. You'll need the PNG image with which you'd like to create a NinePatch image.
- In Android Studio, right-click the PNG image you'd like to create a NinePatch image from, then click Create 9-patch file.
- Type a file name for your NinePatch image, and click OK. Your image will be created with the
.9.png
file extension. - Double-click your new NinePatch file to open it in Android Studio. Your workspace will now open.
The left pane is your drawing area, in which you can edit the lines for the stretchable patches and content area. The right pane is the preview area, where you can preview your graphic when stretched.
- Click within the 1-pixel perimeter to draw the lines that define the stretchable patches and (optional) content area. Right-click (or hold Shift and click, on Mac) to erase previously drawn lines.
- When done, click File >Save to save your changes.
Android Chat Bubble 9 Patch Download Windows 7
You can open an existing NinePatch file in Android Studio by double-clicking the file.
To make sure that your NinePatch graphics scale down properly, verify that any stretchable regions are at least 2x2 pixels in size. Otherwise, they may disappear when scaled down. Also, provide one pixel of extra safe space in the graphics before and after stretchable regions to avoid interpolation during scaling that may cause the color at the boundaries to change.
Note: A normal PNG file (*.png
) will be loaded with an empty one-pixel border added around the image, in which you can draw the stretchable patches and content area. A previously saved NinePatch file (*.9.png
) will be loaded as-is, with no drawing area added, because it already exists.
Figure 2. A NinePatch image showing content, patches, and bad patches.
Optional controls include:
- Zoom: Adjust the zoom level of the graphic in the drawing area.
- Patch scale: Adjust the scale of the images in the preview area.
- Show lock: Visualize the non-drawable area of the graphic on mouse-over.
- Show patches: Preview the stretchable patches in the drawing area (pink is a stretchable patch), as shown in figure 2, above.
- Show content: Highlight the content area in the preview images (purple is the area in which content is allowed), as shown in figure 2.
- Show bad patches: Adds a red border around patch areas that may produce artifacts in the graphic when stretched, as shown in figure 2. Visual coherence of your stretched image will be maintained if you eliminate all bad patches.